머신러닝이 잘 되고 있는지, 결과가 잘 출력되는지 확인하기 위해서는 좋은 시각화 도구가 필요합니다.
matplotlib.pyplot를 이용하여 그래프 그리기에 익숙해지기 위한 간단한 튜토리얼을 진행합니다.
1. 선 그래프
import matplotlib.pyplot as plt
import random
# plot
x = range(20)
y = random.sample(range(0, 20), 20)
plt.plot(x, y)
plt.show()
plt.plot(x, y, 'bo')
plt.show()
plt.plot(x, y, 'r-')
plt.show()
plt.plot(x, y, 'g--')
plt.show()
plt.plot(y, 'k-')
plt.xlabel('X')
plt.ylabel('Y')
plt.title('Title')
x = range(20)
y = random.sample(range(0, 20), 20)
plt.plot(x, y, 'y-')
plt.legend(['Loss', 'Accuracy'])
plt.show()
# subplot
x = range(20)
y = random.sample(range(0, 20), 20)
plt.subplot(1, 2, 1)
plt.plot(x, y, 'g-')
x = range(20)
y = random.sample(range(0, 20), 20)
plt.subplot(1, 2, 2)
plt.plot(x, y, 'r-')
plt.show()
x = range(20)
-> x에 [0, 1, 2, ..., 19]의 20개의 정수로 구성된 리스트를 할당
y = random.sample(range(0, 20), 20)
-> 0부터 19까지의 숫자 중 하나를 가지는 길이가 20인 리스트를 할당 (중복 제외)
plt.plot(x, y)
-> x, y를 기반으로 (꺾은)선 그래프를 그림
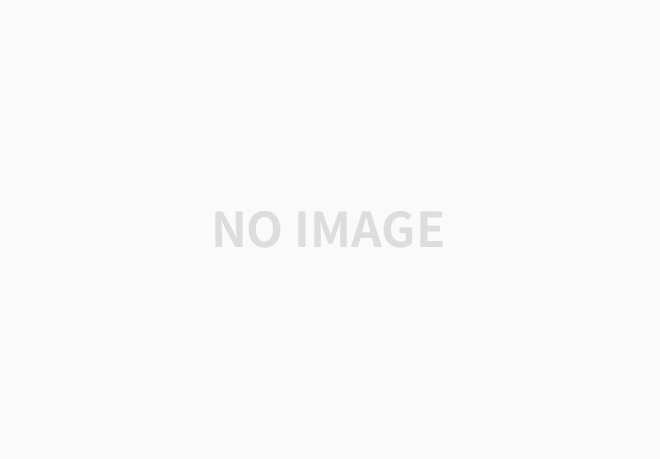
plt.show()
-> 그린 그래프를 화면에 출력
plt.plot(x, y, 'bo')
-> x, y를 기반으로 점으로 그림
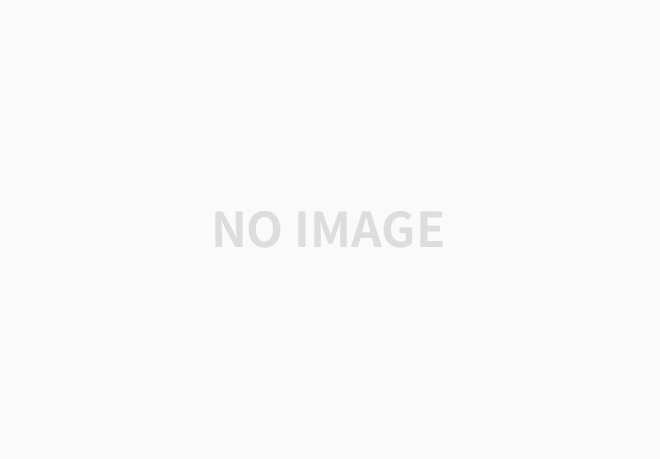
plt.plot(x, y, 'r-')
-> x, y를 기반으로 빨간색 선 그래프를 그림
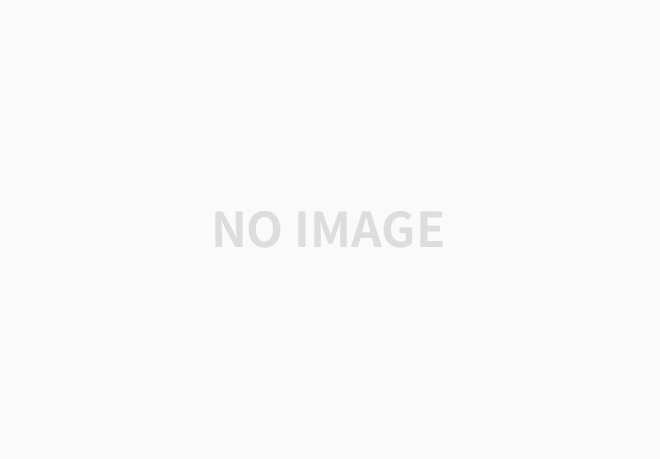
plt.plot(x, y, 'g--')
-> x, y를 기반으로 초록색 점선 그래프를 그림
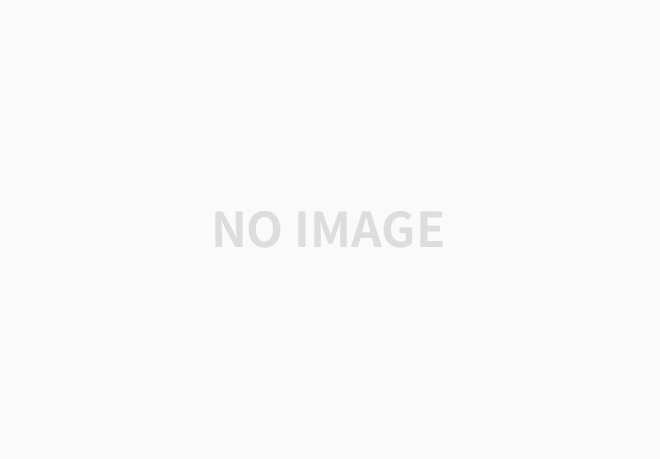
plt.plot(y, 'k-')
-> x값을 전달하지 않으면 x는 자동으로 range(len(y))에 해당하는 값을 할당 후 선 그래프를 그림
plt.xlabel('X')
-> x축 레이블 설정
plt.xlabel('Y')
-> y축 레이블 설정
plt.title('Title')
-> 플롯의 제목 설정
plt.legend(['Loss', 'Accuracy'])
-> 범례 설정
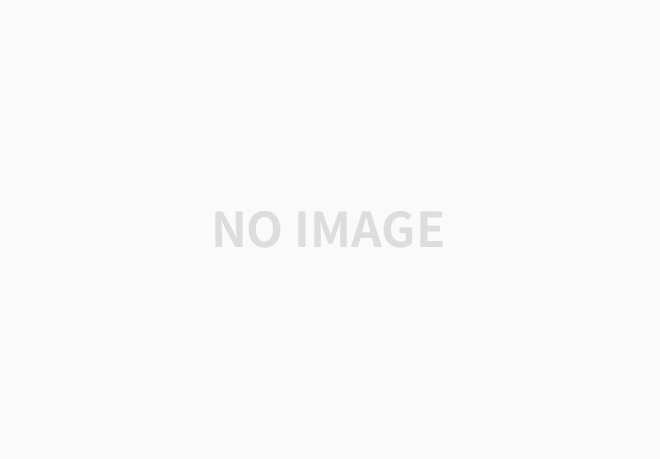
plt.subplot(1, 2, 1)
-> 1행 2열 1번째 플롯
plt.subplot(1, 2, 2)
-> 1행 2열 2번째 플롯
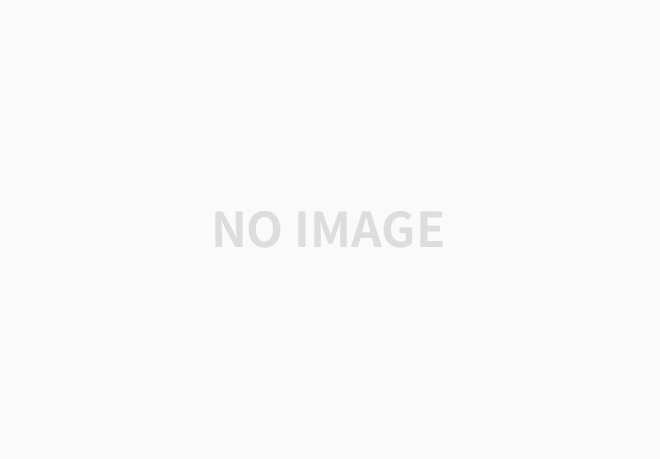
2. 히스토그램
import matplotlib.pyplot as plt
import random
data = [random.randrange(10) for i in range(100)]
plt.subplot(1, 2, 1)
plt.hist(data)
data = [random.randrange(10) for i in range(100)]
plt.subplot(1, 2, 2)
plt.hist(data,
bins=8, ## 몇 개의 바구니로 구분할 것인가.
density=True, ## ytick을 퍼센트비율로 표현해줌
cumulative=False, ## 누적으로 표현하고 싶을 때는 True
histtype='bar', ## 타입. or step으로 하면 모양이 바뀜.
orientation='vertical', ## or horizontal
rwidth=0.8, ## 1.0일 경우, 꽉 채움 작아질수록 간격이 생김
color='hotpink', ## bar 색깔
)
plt.show()
data = [random.randrange(10) for i in range(100)]
-> 0부터 9까지의 숫자 중 하나를 가지는 길이가 100인 리스트를 할당 (중복 허용)
plt.hist(data,
bins=8, ## 몇 개의 바구니로 구분할 것인가.
density=True, ## ytick을 퍼센트비율로 표현해줌
cumulative=False, ## 누적으로 표현하고 싶을 때는 True
histtype='bar', ## 타입. or step으로 하면 모양이 바뀜.
orientation='vertical', ## or horizontal
rwidth=0.8, ## 1.0일 경우, 꽉 채움 작아질수록 간격이 생김
color='hotpink', ## bar 색깔
)
-> 히스토그램 그림
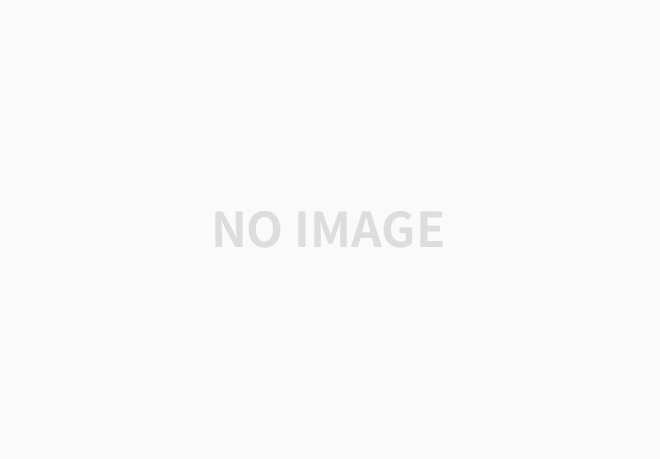
'AI > Machine Learning' 카테고리의 다른 글
[Machine Learning] 선형대수 - 특이값 분해 (0) | 2022.08.09 |
---|---|
[Machine Learning] 선형대수 - 고윳값 분해 (0) | 2022.08.09 |
[Machine Learning] 데이터 전처리 (0) | 2022.07.11 |
[Machine Learning] Sklearn을 이용한 머신러닝 데이터 불러오기 (0) | 2022.07.08 |
[Machine Learning] 머신 러닝이란 (0) | 2021.04.02 |